Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 알고리즘풀이
- 코틀린기초
- binary search
- python zip_longest
- 릿코드풀이
- 릿코드
- python Leetcode
- leetcode풀이
- LeetCode
- 릿코드 풀기
- python 릿코드
- 파이썬 알고리즘 풀기
- 릿코드 파이썬
- 파이썬알고리즘풀기
- 파이썬릿코드풀기
- python 알고리즘
- python priority queue
- 파이썬릿코드
- 파이썬 프로그래머스
- leetcode풀기
- 릿코드풀기
- python sorted
- 파이썬알고리즘
- python xor
- 파이썬 릿코드
- leetcode 풀기
- 파이썬 알고리즘
- 상가수익률계산기
- 잇츠디모
- 알고리즘풀기
Archives
- Today
- Total
소프트웨어에 대한 모든 것
540. Single Element in a Sorted Array 본문
반응형
540. Single Element in a Sorted Array
https://leetcode.com/problems/single-element-in-a-sorted-array/
Single Element in a Sorted Array - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제)
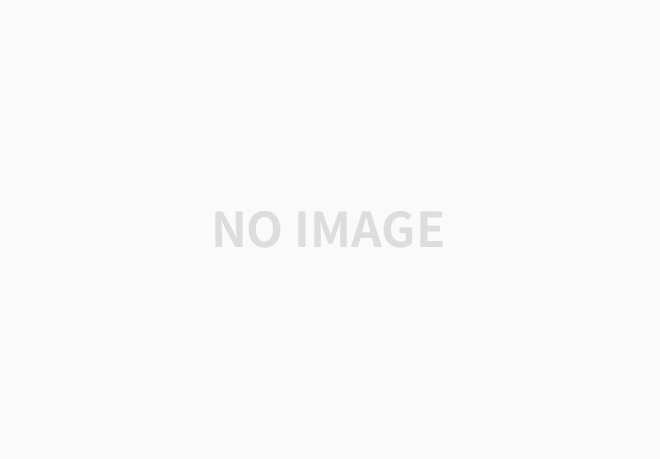
솔루션1) - hash 사용
단 하나의 elemnt를 제외하고 모든 원소들은 두 번 등장합니다.
hash 자료구조에 처음 나오는 element를 저장하고, 두 번째 등장하게 되면 hash에 삭제합니다.
그러면 최종적으로 한 번 등장한 원소만 hash에 남게 됩니다.
시간 복잡도 : O(n)
공간 복잡도 : O(n)
class Solution:
def singleNonDuplicate(self, nums: List[int]) -> int:
# hash
d = {}
for n in nums:
if n in d:
del d[n]
else:
d[n] = None
return list(d.keys())[0]
솔루션2) Counter 객체 사용
시간 복잡도 : O(n)
공간 복잡도 : O(n)
class Solution:
def singleNonDuplicate(self, nums: List[int]) -> int:
counter = Counter(nums)
for n, count in counter.items():
if count == 1:
return n
return None
솔루션3) 모든 원소들은 두 번 등장
단 하나의 elemnt를 제외하고 모든 원소들은 두 번 등장하는 특징을 사용합니다.
반복문을 돌면서 i, i+1 인덱스의 값을 체크하면서 동일하지 않는 경우가 발생하면 그 요소가 한 번 등장한 값이 됩니다.
시간 복잡도 : O(n)
공간 복잡도 : O(1)
class Solution:
def singleNonDuplicate(self, nums: List[int]) -> int:
for i in range(0, len(nums) - 1, 2):
if nums[i] != nums[i+1]:
return nums[i]
return nums[-1]
반응형
'알고리즘 > LeetCode' 카테고리의 다른 글
LeetCode 풀기 - 1380. Lucky Numbers in a Matrix (0) | 2021.12.29 |
---|---|
LeetCode 풀기 - 227. Basic Calculator II (0) | 2021.12.28 |
LeetCode 풀기 - 973. K Closest Points to Origin (0) | 2021.12.26 |
LeetCode 풀기 - 56. Merge Intervals (0) | 2021.12.24 |
LeetCode 풀기 - 143. Reorder List (0) | 2021.12.23 |