Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- python zip_longest
- 알고리즘풀기
- 릿코드 파이썬
- 릿코드 풀기
- binary search
- 파이썬 알고리즘 풀기
- 파이썬 알고리즘
- 릿코드풀기
- 파이썬 프로그래머스
- 파이썬릿코드풀기
- LeetCode
- leetcode풀기
- 잇츠디모
- 파이썬알고리즘풀기
- python 알고리즘
- python xor
- 릿코드
- leetcode풀이
- python sorted
- 릿코드풀이
- 알고리즘풀이
- 파이썬 릿코드
- leetcode 풀기
- python priority queue
- python Leetcode
- python 릿코드
- 상가수익률계산기
- 파이썬릿코드
- 코틀린기초
- 파이썬알고리즘
Archives
- Today
- Total
소프트웨어에 대한 모든 것
[디자인패턴][Composite] 컴포지트 패턴 본문
반응형
컴포지트 패턴 정의
컴포지트 패턴(합성 패턴)은 하나의 클래스와 복합 클래스를 동일한 구성을 하여 사용하는 방법입니다. 컴포지트 패턴은 디렉토리, 파일과 같은 트리 구조의 재귀적 데이터 구조를 표현하는데 적합한 패턴입니다. 컴포지트 의도는 트리 구조로 작성하여, 전체-부분(whole-part) 관계를 표현하는 것입니다.
컴포지트 패턴 언제 사용
- 전체-부분 관계를 트리 구조로 표현하고 싶을 경우
- 전체-부분 관계를 클라이언트에서 부분, 관계 객체를 동일하게 처리하고 싶은 경우
컴포지트 패턴 클래스 다이어그램
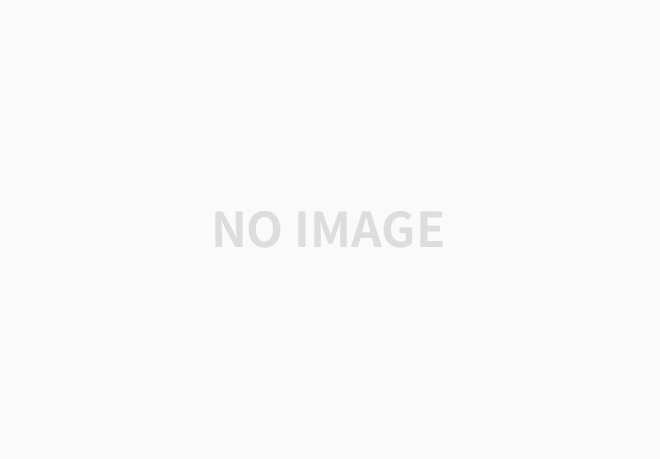
- Client는 Lean와 Composite를 직접 참조 하지 않고 공통 인터페이스 Componet를 참조
- Leaf는 Component 인터페이스를 구현
- Composite 클래스는 Component 객체 자식들을 유지하고 operation()통해서 작식들에게 전달
컴포지트 패턴 활용
파일 시스템은 대표적은 트리 구조 형태를 가집니다. 디렉토리가 있고 디렉토리 내에서 파일이 있고 또 다른 디렉토리가 있을 수 있습니다. 트리구조 이면서 전체-부분의 형태를 가집니다. 컴포지트 패턴을 적용하기에 아주 적합합니다.
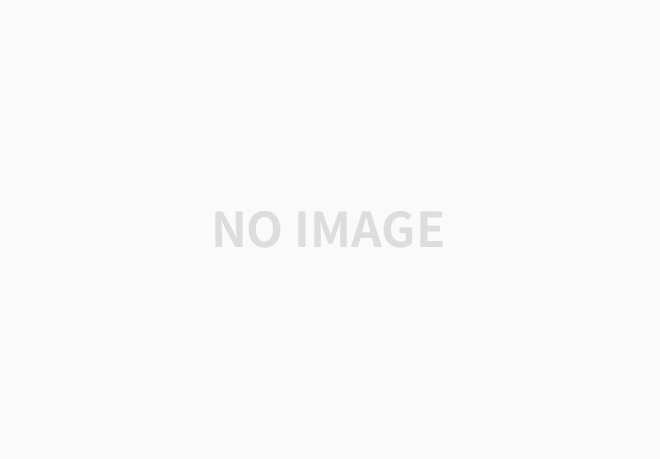
- Node는 추상 클래스
- File은 Node를 상속받은 클래스 (leaf class)
- Directory는 Node를 상속받고 Node 관리하기 위한 nodes가 있습니다. 클래스 다이어그램에서 합성을 통해서 표시합니다. Node 관리용 add(), remove() 함수도 지원합니다.
from abc import ABC, abstractmethod
class Node(ABC):
def __init__(self):
self.name = None
@abstractmethod
def print(self):
pass
class File(Node):
def __init__(self, name):
self.name = name
def print(self):
print(f'file name : {self.name}')
class Directory(Node):
def __init__(self, name):
self.name = name
self.nodes = []
def add(self, node):
self.nodes.append(node)
def remove(self, node):
self.nodes.remove(node)
def print(self):
print(f'directory name : {self.name}')
for node in self.nodes:
node.print()
# 루트 디렉토리 생성 후 파일 2개 추가
directory = Directory('/')
directory.add(File('a.txt'))
directory.add(File('b.txt'))
# hello 디렉토리 생성 후 파일을 하나 생성하고 루트 디렉토리에 추가
second_directory = Directory('hello')
second_directory.add(File('word.txt'))
directory.add(second_directory)
print('\n루트 디렉토리 출력')
directory.print()
print('\nhello 디렉토리 삭제')
directory.remove(second_directory)
print('\n루트 디렉토리 출력')
directory.print()
출력
루트 디렉토리 출력
directory name : /
file name : a.txt
file name : b.txt
directory name : hello
file name : word.txt
hello 디렉토리 삭제
루트 디렉토리 출력
directory name : /
file name : a.txt
file name : b.txt
Process finished with exit code 0
참고 자료
https://en.wikipedia.org/wiki/Composite_pattern
https://mygumi.tistory.com/343
https://refactoring.guru/design-patterns/composite
반응형
'시스템 설계 및 디자인 > 디자인 패턴' 카테고리의 다른 글
[디자인패턴][Mediator] 중재자 패턴 (0) | 2022.03.24 |
---|---|
[디자인패턴][Observer] 옵저버 패턴 (0) | 2022.03.24 |
[디자인패턴][Prototype] 프로토타입 패턴 (0) | 2022.03.24 |
[디자인패턴][Proxy] 프록시 패턴 (0) | 2022.03.24 |
[디자인패턴][Factory Method] 팩토리 메소드 패턴 (0) | 2022.03.24 |