Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 |
Tags
- 파이썬알고리즘풀기
- leetcode풀기
- 파이썬알고리즘
- python 릿코드
- 잇츠디모
- 릿코드 풀기
- leetcode 풀기
- python zip_longest
- python xor
- 릿코드풀이
- 릿코드풀기
- 파이썬릿코드풀기
- 파이썬 알고리즘 풀기
- 파이썬 릿코드
- leetcode풀이
- python Leetcode
- python sorted
- python 알고리즘
- LeetCode
- python priority queue
- 릿코드 파이썬
- 알고리즘풀이
- binary search
- 알고리즘풀기
- 코틀린기초
- 파이썬 알고리즘
- 상가수익률계산기
- 릿코드
- 파이썬 프로그래머스
- 파이썬릿코드
Archives
- Today
- Total
소프트웨어에 대한 모든 것
LeetCode 풀기 - 70. Climbing Stairs 본문
반응형
70. Climbing Stairs
https://leetcode.com/problems/climbing-stairs/
Climbing Stairs - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제)
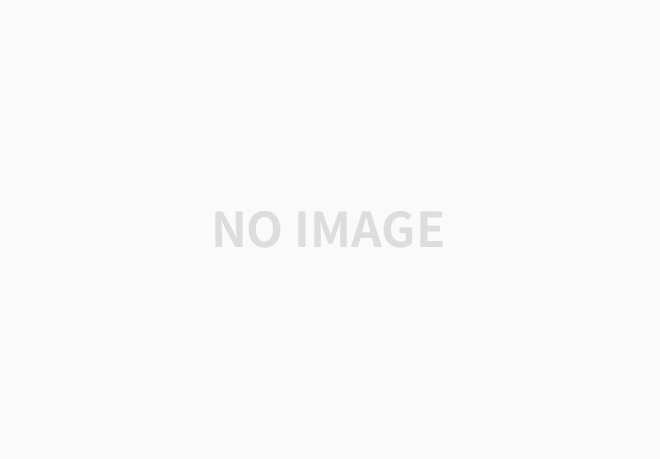
솔루션1)
- 동적 계획법 풀이
class Solution:
def climbStairs(self, n: int) -> int:
'''
f(0) = 0
f(1) = 1
f(2) = 2
f(3) = f(2) + f(1)
...
f(n) = f(n-1) + (n-2)
'''
fn = [0, 1, 2]
# bottom up
for i in range(3, n+1):
fn.append(fn[i-1] + fn[i-2])
return fn[n]
솔루션2)
class Solution:
def climbStairs(self, n: int) -> int:
if n <=2:
return n
a, b, c = 0, 1, 2
# bottom up
for i in range(3, n+1):
a, b = b, c
c = a + b
return c
솔루션3)
- 백트래킹 + 메모이제이션
class Solution:
@lru_cache(maxsize=128)
def climbStairs(self, n: int) -> int:
# backtracking
if n == 0 or n == 1:
return 1
return self.climbStairs(n-1) + self.climbStairs(n-2)
반응형
'알고리즘 > LeetCode' 카테고리의 다른 글
LeetCode 풀기 - 1816. Truncate Sentence (0) | 2021.11.05 |
---|---|
LeetCode 풀기 - 1913. Maximum Product Difference Between Two Pairs (0) | 2021.11.05 |
LeetCode 풀기 - 380. Insert Delete GetRandom O(1) (0) | 2021.11.03 |
LeetCode 풀기 - 1832. Check if the Sentence Is Pangram (0) | 2021.11.03 |
LeetCode 풀기 - 155. Min Stack (0) | 2021.11.02 |
Comments