Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 파이썬알고리즘풀기
- python priority queue
- python 알고리즘
- leetcode풀기
- LeetCode
- python zip_longest
- 알고리즘풀기
- 파이썬알고리즘
- 파이썬릿코드
- 잇츠디모
- 파이썬 알고리즘
- 파이썬 알고리즘 풀기
- 알고리즘풀이
- leetcode풀이
- 릿코드 파이썬
- 릿코드
- 릿코드풀이
- leetcode 풀기
- 코틀린기초
- 파이썬릿코드풀기
- 릿코드풀기
- python sorted
- python 릿코드
- 상가수익률계산기
- python xor
- 파이썬 릿코드
- 릿코드 풀기
- python Leetcode
- binary search
- 파이썬 프로그래머스
Archives
- Today
- Total
소프트웨어에 대한 모든 것
LeetCode 풀기 - 890. Find and Replace Pattern 본문
반응형
890. Find and Replace Pattern
https://leetcode.com/problems/find-and-replace-pattern/
Find and Replace Pattern - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제)
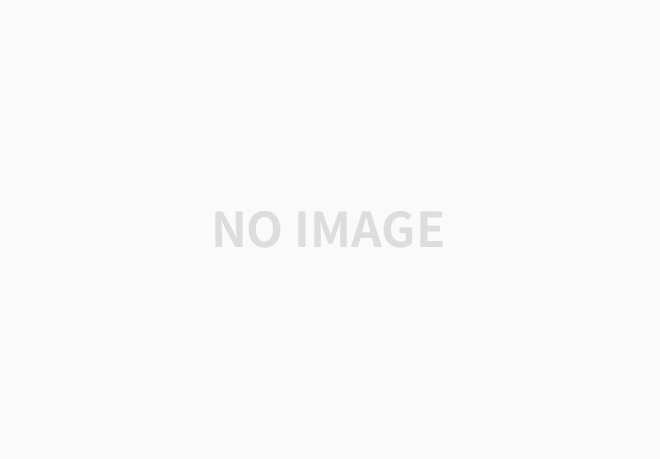
솔루션1)
class Solution:
def findAndReplacePattern(self, words: List[str], pattern: str) -> List[str]:
matched = []
unique_char_count = len(set(list(pattern)))
for word in words:
if len(set(list(word))) != unique_char_count:
continue
# char 매핑
mapping = {}
# 매핑에 의해서 생성된 단어
created_word = ''
for i, c in enumerate(word):
if c in mapping:
# 이미 매핑되어 있다면 사용
created_word += mapping[c]
else:
# 매핑되어 있지않으면 새로운 매핑을 추가
mapping[c] = pattern[i]
created_word += pattern[i]
# 매핑에 의해성 생성된 단어가 패턴가 동일한가?
if created_word == pattern:
matched.append(word)
return matched
솔루션2)
dict의 setdefault() 함수를 이용한다.
매핑 테이블을 만들고 숫자로 변환된 값이 동일한지 체크한다.
예시)
pattern : "abb" -> 011
word1 : "abc" -> 012 (not matched)
word2 : "aba" -> 010 (not matched)
word3 : "mee" -> 011 (matched)
class Solution:
def findAndReplacePattern(self, words: List[str], pattern: str) -> List[str]:
def mapping(word):
m = {}
return [m.setdefault(c, len(m)) for c in word]
pattern_mapping = mapping(pattern)
return [word for word in words if mapping(word) == pattern_mapping]
반응형
'알고리즘 > LeetCode' 카테고리의 다른 글
LeetCode 풀기 - 278. First Bad Version (0) | 2021.11.10 |
---|---|
LeetCode 풀기 - 704. Binary Search (0) | 2021.11.10 |
LeetCode 풀기 - 441. Arranging Coins (0) | 2021.11.09 |
LeetCode 풀기 - 260. Single Number III (0) | 2021.11.09 |
LeetCode 풀기 - 1732. Find the Highest Altitude (0) | 2021.11.09 |